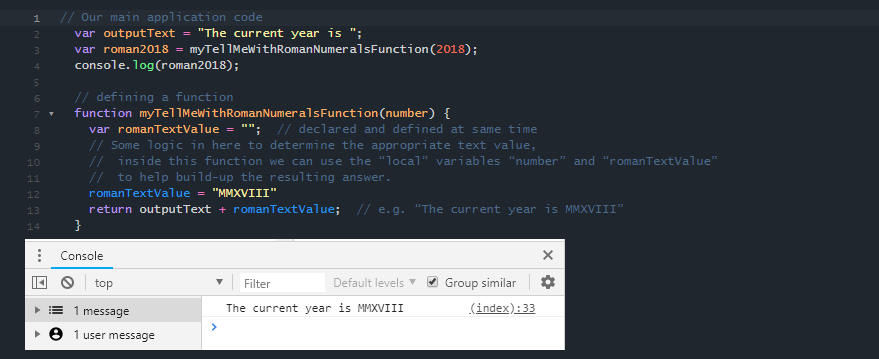
Scope and Javascript
Functions and Variables: Global and Local scope
by: Peter Torr Smith
4th October, 2018
Tags/Categories
Explain to a non-tech friend:
What 'scope' is, and how it works in JavaScript
In programming (Javascript and most other languages), a variable is simply a "thing" (or Object) declared (created) with a name (e.g. "firstName") and an optional defined value (e.g. "Peter").
Scope, in the context of computer programming, is basically the level or range of visibility that a given variable has.
Common terms for defining scope are "local" and "global".
Here’s a non-programming analogy:
Think of it (a little bit) like comparing your local home Wi-Fi with the Cellphone coverage you have.
Your home Wi-Fi lives inside your home/property, and is for the purposes of being available for use within your home/property. When you go into town, that Wi-Fi is no longer available. So we can say that the scope of your home Wi-Fi is "local" to your home/property.
Your cellphone network however is created and managed outside your home, and is available to you not only when you are away from your home, but also within your home, when you are in someone else’s home, out walking in the park, or even visiting another city (in NZ). So we can say that the scope of the cellphone network is "global" (well at least within most of Aotearoa NZ), in that it is available outside and inside your home.
So in regards to programming?
In programming, application logic in code is usually broken into small chunks of code ("functions") that do specific things, instead of one big long block of code. This could be a "function" which takes a number as input and returns some text to display using the roman-character equivalent of the number provided. We could then use that function in our main application code:
// Our main application code
var outputText = "The current year is "; // global scope
var roman2018 = myTellMeWithRomanNumeralsFunction(2018); // global scope
console.log(roman2018); // or use otherwise to display in your web application
// defining a function
function myTellMeWithRomanNumeralsFunction(number) {
var romanTextValue = ""; // scope is local this only within this function
// Some logic in here to determine the appropriate text value,
// inside this function we can use the "local" variables "number" and "romanTextValue"
// to help build-up the resulting answer.
romanTextValue = "MMXVIII"
return outputText + romanTextValue; // e.g. "The current year is MMXVIII"
}
// end of defining the function
In the above code, a function "myTellMeWithRomanNumeralsFunction" has been defined to take an input "number", do some things, and return a value.
Outside of the function, whilst our outer code can call the function ( var roman2018 = myTellMeWithRomanNumeralsFunction(2018)
) to get and assign the resulting value to the variable roman2018 ("The current year is
MMXVIII"), our outer code cannot reference, use, see or change any of the functions "local"
variables (ie. "number" and "romanTextValue"), as they have local scope... local only to
within the function they are declared in.
However inside the function, the function can access, use and update any "global" variables, that are defined outside that function (in this case, it can see and use the variable "outputText" as it has global scope.